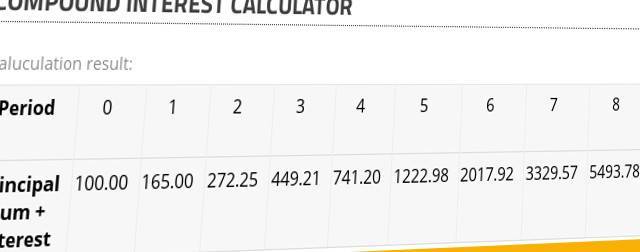
Compound interest in PHP part 2
Created:21 Sep 2017 12:43:34 , in Host development
First article ( a link to it can be found at the end of this article ) in this series was all about developing libraries for compound interest calculation in PHP programming language. Now, it is time to employ those pieces of code to do what they have been built for.
In this article I introduce a CLI script and a web form for finding compound interest and total accumulated value on a principal sum.
Basic tools for developing command line scripts
Largely, building a command line program in PHP is similar to doing it in C programming language ( or perhaps BASH if C is not your cup of tea anymore ). You get access to standard input, output and error streams, you meet old friends argv and argc (they are global variables in PHP), you even have that (in)famous getopt function ( or its better, modern incarnation ) at your disposal.
Standard input, standard output, and standard error can be accessed through STDIN, STDOUT and STDERR constants respectively. No opening or closing these streams is necessary.
Argc and argv tell you how many and what arguments are passed to your script respectively. The latter, as is customary, holds your script name as its first argument. No surprises here.
Getopt allows to collection of parameters from the command line in PHP. This function deals smoothly with picking up optional and required values for configured parameters as well as handling script parameters which do not accept a value.
On this occasion two things worth noting with regards to getopt are:
-
When a parameter is not present on the command line there is no trace of it in the array getopt returns either.
A parameters which does not require a value still gets it in the associative array getopt returns. The value is boolean false.
Getopt is a perfect tool for overwriting defaults in a script with user supplied data (take a look at the first part of this series for a way it can be done).
Both good number of examples and generally a lot of details on getopt and I/O streams are available on exquisitely documented PHP website. Check a links section at the end of this article.
Command line compound interest script
Broadly, my compound interest CLI script consists of two parts. In the first required libraries get loaded ( for simplicity it is assumed they are all in the script directory).
The second is a PHP class which contains a public method called "init". The method contains logic responsible for the script short name command line arguments configuration (getopt), user input validity check, and outputting a calculation result to standard output stream (or error and help messages to standard error and standard output stream respectively if user supplied data was invalid).
Here is the script:
#!/usr/bin/env php
<?php
require_once('CompoundInterestBase.php');
require_once('CompoundInterestValid.php');
require_once('SWWWValidation.php');
require_once('SWWWStringArrayFormat.php');
require_once('SWWWCompoundInterest.php');
class SWWWCompoundInterestRunner{
private static function help(){
global $argv;
$script_name = basename($argv[0]);
$usage = "Usage: {$script_name} options\n";
$usage .= " options:\n";
$usage .= " -p - principal sum\n";
$usage .= " -r - interest rate\n";
$usage .= " -f - frequency of compounding\n";
$usage .= " -y - years of compounding\n";
$usage .= " [ -g ] - interest accumulated only\n";
$usage .= " [ -n ] - display all sums as opposed to the last one only\n";
$usage .= " [ -t ] - format - as accepted by sprintf PHP function\n";
return $usage;
}
// return first found error
private static function error($errors){
foreach($errors as $opt => $messages){
return "Error: {$errors[$opt][0]} \n";
}
}
// script entry point function
public static function init(){
// command line options
$conf = getopt('p:r:f:y:t:ng');
$output = null;
$instance = new SWWWCompoundInterest($conf);
if($instance -> valid()){
$output = $instance -> get();
if( is_array($output)){
$output = implode(',',$output);
}
fwrite(STDOUT,$output);
}else{
fwrite(STDERR,self::error($instance -> error()));
fwrite(STDOUT,self::help());
}
}
}
// run this script
SWWWCompoundInterestRunner::init();
?>
Compound interest in the browser
As mentioned earlier in this article, apart of the CLI script I also built a web browser accessible compound interest calculator.
Final thoughts
PHP is considered a capable programming language for building web applications, but it also provides very decent tools for building command line scripts. Getopt function and solutions for handling I/O streams are just basic examples of those.
Links
getopt PHP function
argv PHP global variable
I/0 streams in PHP
This post was updated on 04 Nov 2017 11:33:21
Tags:
php
Author, Copyright and citation
Author
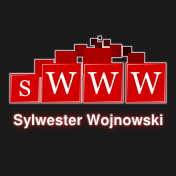
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2025 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. " Compound interest in PHP part 2." From sWWW - Code For The Web . https://swww.com.pl//main/index/compound-interest-in-php-part-2
Add Comment