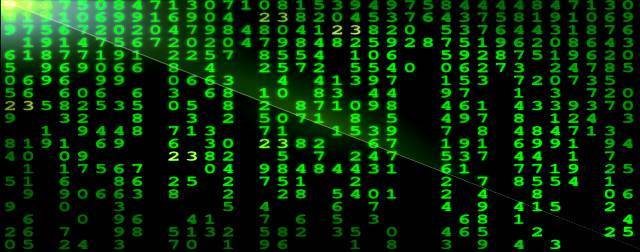
Matrix transpose operator implementation in PHP
Created:05 Oct 2017 21:49:26 , in Maths
If you look for a computer programming challenge, you'll certainly find a few decent ones implementing concepts Linear Algebra deals with.
One of simpler operations, at least on the paper, one carries out on the matrix is switching rows and columns indices along the main diagonal of it. In Linear Algebra the operator which does this exact thing is called the transpose of a matrix. In this article I look how to implement this operator in PHP programming language.
Even though, transposing a matrix is a common and frequently unavoidable operation in Linear Algebra, doing it on anything beyond 2 x 2 matrix more than once or twice by hand is an a boring, error prone and time consuming task. You are better off investing your time in writing your own matrix transposing function (or perhaps learning how to use an already existing piece of matrix transposing software) than doing that.
In short, transposing matrices is a challenge, which is good for computers and computers only.
Flavors of matrices to transpose
The transpose of a matrix is a unary operator which accepts a matrix and returns a (transposed) matrix. The input matrix can be either a square or a rectangular one. The former has the same count of rows and columns, the latter can have either more rows or more columns. All three possibilities will have to be taken into account in the code.
Transpose of transpose of a matrix is the input matrix
The transpose of the matrix operator has some nice properties. One of them is that a matrix trasposed twice yields the original matrix. This is property can serve as a good quality check for a matrix transpose operator implementation.
Matrix transpose implementation
My implementation of the transpose of a matrix consists of just one one static method which sits in SWWWMatrix PHP class.
The code is somewhat dense here and there so I've left my comments in tact:
/*
Class: SWWWMatrix
Description: Conduct operations on a matrix
Author: Sylwester Wojnowski
WWW: wojnowski.net.pl
Methods:
transpose($A) - public static - transpose a matrix
parameters:
$A - array - representation of a matrix
return - array - representation of a transposed matrix
*/
class SWWWMatrix{
public static function transpose($A){
// reset all the variables before they are used
$i = $j = $k = $l = $m = null;
// set number of rows
$i = count($A);
// return if there are no rows
if($i === 0){ return $A; }
// set number of columns
$j = count($A[0]);
// handle matrices with fewer rows than columns or the same number of rows and columns
if($i <= $j){
// add extra rows to the end of the matrix if necessary
for($k = 0; $k < $j - $i; $k++){
$A[$i + $k] = array();
}
// switch indices of rows and columns in the matrix
for($l = 0; $l < $i; $l++){
for($m = $j; $m > $l; $m--){
if(!isset($A[$m - 1][$l])){
$A[$m - 1][$l] = $A[$l][$m - 1];
unset($A[$l][$m - 1]);
}else{
$temp = $A[$m - 1][$l];
$A[$m - 1][$l] = $A[$l][$m - 1];
$A[$l][$m - 1] = $temp;
}
}
}
// handle matrices with more rows than columns
} else {
// switch indices of rows and columns in the matrix
for($l = 0; $l < $i; $l++){
for($m = 0; $m < $j && $m < $l; $m++){
if(!isset($A[$m][$l])){
$A[$m][$l] = $A[$l][$m];
unset($A[$l][$m]);
if(empty($A[$l])){ unset($A[$l]);}
} else {
$temp = $A[$m][$l];
$A[$m][$l] = $A[$l][$m];
$A[$l][$m] = $temp;
}
}
}
}
// we are done, return transposed matrix
return $A;
}
}
SWWWMatrix::transpose works on the same matrix it accepted as its input. Also, SWWWMatrix::transpose works on array indices, so you can store whatever objects you like in your matrix.
Use examples
As the first example a rectangular matrix with fewer rows than columns gets transposed:
$A = array(
array(1,3,5),
array(2,4,6)
);
$ATrans = SWWWMatrix::transpose($A);
/*
=>
$ATrans = array(
array(1,2),
array(3,4),
array(5,6)
);
*/
Here is a slightly different rectangular matrix:
$A = array(
array(1,2),
array(3,4),
array(5,6)
);
$ATrans = SWWWMatrix::transpose($A);
/*
=> $ATrans = array(
array(1,3,5),
array(2,4,6)
);
*/
Transposing 5 x 5 square matrix:
$A = array(
array( 1, 2, 3, 4, 5),
array( 6, 7, 8, 9,10),
array(11,12,13,14,15),
array(16,17,18,19,20),
array(21,22,23,24,25)
);
$ATrans = SWWWMatrix::transpose($A);
/*
=>
$ATrans = array(
array( 1, 6,11,16,21),
array( 2, 7,12,17,22),
array( 3, 8,13,18,23),
array( 4, 9,14,19,24),
array( 5,10,15,20,25)
);
*/
You are not limited to these small matrices. The function can handle much larger ones.
Final thoughts
Linear Algebra is an awesome subject to study, it becomes even more interesting when one gets beyond the usual theory and arithmetic it is comprised of and extends it by implementations of some of the concepts in a programming language of choice.
I hope this article both is a nice introduction to this extended way of learning Maths and a source of a useful piece of code.
This post was updated on 05 Oct 2017 21:57:56
Tags:
matrix
Author, Copyright and citation
Author
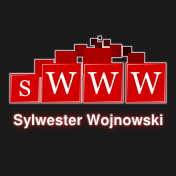
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2024 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. "Matrix transpose operator implementation in PHP." From sWWW - Code For The Web . https://swww.com.pl//main/index/matrix-transpose-operator-implementation-in-php
Add Comment