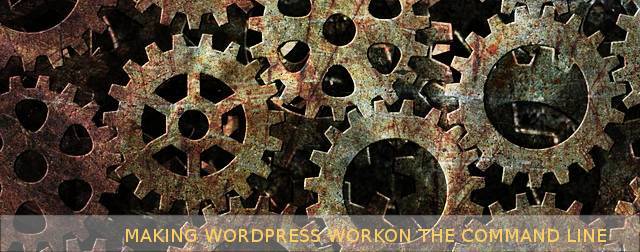
Using WordPress on the command line
Created:20 Apr 2018 15:12:07 , in Web development
There are situations where it is handy to speak to WordPress directly from the the command line. For example, imagine that you must regain access to your website's admin panel after you have your website's admin area access locked out because you have forgotten your password or perhaps because you've been hacked and your login and password have been changed by the attacker.
Sometimes you just need to run some one-off server job which would both require little effort to write and work great if given access to functionality available in WordPress. Administrative tasks and database data manipulations are common examples here.
For these and tons of similar cases often a good solution seems to be a quick command line utility in PHP which will make use of some WordPress API to perform required task efficiently. However, these APIs are available to your command line script only after WordPress environment has been properly initialized.
In this article I explore how to put WordPress to work on the command line. As parts of it I include a tiny PHP class which initializes WordPress environment and another one which provides some basic but useful functionality. Both are meant to give you an idea regarding one way of scripting common tasks for your WordPress blog or website.
Initializing WordPress environment in a PHP script
Initializing WordPress environment in a PHP script is a relatively uncomplicated task. You do it through including wp-load.php file and then letting WordPress know it should load no theme.
Below is a PHP class called WPCliLoad, which performs these two steps.
/*
Class: WPCliLoad - initialized WordPress environment
Author : Sylwester Wojnowski
WWW : wojnowski.net.pl
*/
class WPCliLoad{
protected $scriptname = NULL;
function __construct($conf){
$this -> scriptname = basename(__FILE__);
if( php_sapi_name() !== 'cli'){
# exit if this script is not being run from the command line
exit($this -> scriptname .' is meant to be run from the command line!');
}
if(!isset($conf['basedir'])){
# exit if base dir is not a WordPress installation root directory
exit('WordPress root directory not given!');
}
$basedir = $conf['basedir'];
if(! is_dir($conf['basedir']) || ! is_file($conf['basedir'] . '/wp-load.php')){
# exit if base dir is not a WordPress installation root directory
exit($basedir .' is not a root directory of WordPress!');
}
# do not load themes
define('WP_USE_THEMES', false);
# load WordPress
require_once($basedir . '/wp-load.php');
}
}
Interacting with WordPress on the command line
Once WordPress environment is initialized, one can start interacting with database, e-mail or wp-cron systems. I provide some samples of creating these interactions in PHP class called WPCliUtils.
WPCliUtils is an extension of WPCliLoad. It consists of public methods get_wp_version, send_email_from_admin, and reset_user_password. As you might have guessed by now, get_wp_version() obtains WordPress installation version for you.
Send_email_from_admin() method allows for sending basic e-mail messages from your server with sender of them being administrator of the website.
Finally, reset_user_password() finds a user by their e-mail address in the database and assigns a new password to them. This method can be used to reset password for both the administrator and the ordinary registered user.
/*
Class: WPCliUtils - WordPress command line utilities
Author : Sylwester Wojnowski
WWW : wojnowski.net.pl
Methods:
public get_wp_version()
Description:
obtain WordPress installation version
return:
(string) version number.
public send_email_from_admin($to, $subject, $message)
Description:
send e-mail from admin of website
Parameters:
$to (string) adresee e-mail address
$subject (string) e-email subject
$message (string) e-mail message
return:
(bool) true on success false on failure
public reset_user_password($email,$password)
Description:
reset WordPress user password
Parameters:
$email (string) e-mail address of user to change password for
$password (string) new plain text password
return:
(bool) - true on success false on failure
*/
class WPCliUtils extends WPCliLoad{
function __construct($conf){
parent::__construct($conf);
}
# get WordPress installation version
public function get_wp_version(){
global $wp_version;
return $wp_version;
}
# send an email from admin to somebody
public function send_email_from_admin($to, $subject, $message){
global $wpdb;
$name = '';
$name_set = $wpdb->get_results( "SELECT `user_nicename` FROM {$wpdb->prefix}users where ID = 1", OBJECT );
$email = get_bloginfo('admin_email');
if( count($name_set) !== 1 or ! $email ){
return false;
}
#do basic data cleanup
$name = sanitize_text_field($name_set[0] -> user_nicename);
$email = sanitize_email($email);
$to = sanitize_email($to);
$subject = sanitize_text_field($subject);
# sanitize_textarea_field() available since v 4.7.0
$message = sanitize_textarea_field($message);
#send e-mail
return wp_mail(
$to,
$subject,
$message,
array('From: '.$name.' < '.$email.' >')
);
}
# Resetting WordPress user password from the command line
public function reset_user_password($email,$password){
## get user if exists
$updated = NULL;
global $wpdb;
$sql = $wpdb -> prepare("select `ID` from {$wpdb->prefix}users where user_email = %s",$email);
$ID_set = $wpdb->get_results($sql, OBJECT );
if( count($ID_set) !== 1 ){
return false;
}
$updated = $wpdb -> update(
"{$wpdb->prefix}users",
array('user_pass' => wp_hash_password($password)),
array('ID' => $ID_set[0] -> ID)
);
return ($updated === 1) ? true : false;
}
}
Usage
Using WPCliLoad and WPCliUtils is very straightforward. You just need to include both classes in your script, pass full path to a directory wp-load.php is in (the file is in the root directory of your WordPress installation together with wp-config.php and index.php files ) as a configuration parameter, initialize WPCliUtils and call your method of interest.
#configure
$wpcliutils_conf = array(
'basedir' => '/full/path/to/wp/root/directory'
);
#instantiate
$wpu = new WPCliUtils($wpcliutils_conf);
# get WordPress installation version
$wpu -> get_wp_version();
# send email from admin
$wpu -> send_email_from_admin('adresee@domain.example','Subject','E-mail message');
# set new password for user identified by user@domain.example
$wpu -> reset_user_password('user@domain.example','new-password');
Final thoughts
As it turns out, WordPress works well not only through a server API but also as a part of a command line script. For these of you who do not want or have no time for writing their own website administration scripts a good news is a lot of common tasks have been automated already and are available through excellent wp-cli utility.
I hope you'have enjoyed the article and more importantly learnt something useful from it.
This post was updated on 20 Apr 2018 15:13:57
Author, Copyright and citation
Author
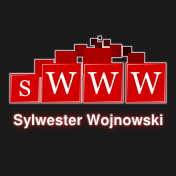
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2024 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. "Using WordPress on the command line." From sWWW - Code For The Web . https://swww.com.pl//main/index/using-wordpress-on-the-command-line
Add Comment