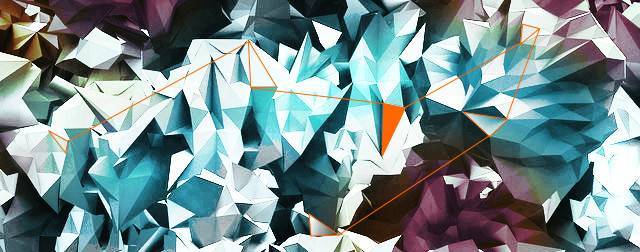
Non-destructive array extending in PHP
Created:25 May 2017 13:30:48 , in Web development
Insert an item into an array without overwriting another item.
/*
ArrayInsert::itemAt
Description: non-destructive extending of PHP number-indexed array
Author: Sylwester Wojnowski
WWW: wojnowski.net.pl
paremeters:
$arr - number-indexed Array
$k - index to insert $item in $arr
$item - piece of data to be inserted in $arr
return value
Extended array
*/
class ArrayInsert{
public static function itemAt($arr,$k,$item){
$j = 0;
$n = count($arr);
if($k >= $n){
$arr[] = $item;
return $arr;
}
while( $j < $n ){
if($j === $k){
$i = -1;
# items at the indices >= to $k have to be moved to the right
while($i + $n >= $j ){
$arr[ $i + $n + 1 ] = $arr[$i + $n];
if($i + $n === $j){
$arr[$j] = $item;
break;
}
$i--;
}
}
$j++;
}
return $arr;
}
}
Some calls below:
Inserting a string in the middle of array:
ArrayInsert::itemAt(array('a','c'),1,'b')
=> array('a','b','c')
Inserting an array in the middle of another array
ArrayInsert::itemAt(array('absinth','sake'),1,array('bitter ale','cask ale','mead'))
=> array('absinth',array('bitter ale','cask ale','mead'),'sake')
Inserting an object at the beginning of array:
$guitars = array(
(object)array(
'brand' => 'Jackson',
'model' => 'King V'
),
(object)array(
'brand' => 'Gibson',
'model' => 'Les Paul Studio'
),
(object)array(
'brand' => 'Schecter',
'model' => 'Hellriser C1'
)
);
$bc_rich = (object)array('brand' => 'BC Rich', 'model' => 'Warlock');
$guitars = ArrayInsert::itemAt($guitars,0,$bc_rich);
Guitars array has 4 items now, with the first items brand property value being "BC Rich".
Inserting NULL at a particular index.
ArrayInsert::itemAt(array('a',null),0,null)
=> array(null,'a',null)
Inserting multiple items in an array non-destructively
Now suppose you need to insert an array of items into your current array with first of the items to be put in at some particular index and the rest behind it.
One way to do that is by adding method "flatten" to ArrayInsert class. Here is a listing of this method:
public static function flatten($arr){
return iterator_to_array(
new RecursiveIteratorIterator(new RecursiveArrayIterator($arr))
,false);
}
And here is an example of use of "flatten" and "arrayAt" together:
# inserting an array of strings with the first of them being added at index 1
ArrayInsert::flatten(
ArrayInsert::itemAt(array(),1,array('red','blue',array('orange','pink'),'green')))
);
=> array('red','blue','orange','pink','green')
This post was updated on 06 Oct 2021 21:28:56
Tags:
php
Author, Copyright and citation
Author
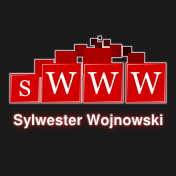
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2024 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. "Non-destructive array extending in PHP." From sWWW - Code For The Web . https://swww.com.pl//main/index/non-destructive-array-extending-in-php
Add Comment