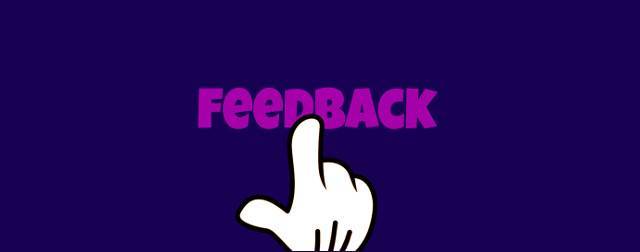
Mapping numeric score to feedback message in PHP
Created:06 Sep 2017 23:04:22 , in Web development
The other day I coded a sort of game in PHP programming language. One of it's features is a final score pop-up message, which consists of three parts. They are a number, a percent and a few words of a humoristic player performance feeback. The feedback is based upon the number or the percent the player scores.
When I first looked at how to design that sort of functionality, I thought, a simple mapping from a number to a string would suffice.
You provide some feedback in the form of a string for lets say score of 20%. When the score is 20%, a player is shown the message. There is a small trouble with this solution, though. In all but the simplest cases, the score can be anything between 0% and 100% inclusive, perhaps 19.53% or 20.0091% instead of 20%, you have prepared a feedback for. It looks like the basic idea misfires for all but few cases here.
A better, functional design is not far away, though. It is based on the idea of mapping intervals rather than numbers to strings. In short, SWWWScoreFeedback, a tiny PHP programming language class, does exactly this sort of thing.
Here is the code:
/*
Class: SWWWScoreFeedback
Description: Get feedback based on score
Author: Sylwester Wojnowski
WWW: wojnowski.net.pl
Methods:
instnce($conf) - public static - get instance of SWWWScoreFeedback
parameters:
$conf - array - associative array of scores/messages
return - object - instance of SWWWScoreFeedback
get($score) - public - get feedback message
parameters:
$score - number|string - number or percent in range 0 - 100 inclusive
[$func] - function - callback function
return - string - feedback for the score
*/
class SWWWScoreFeedback{
const NO_FEEDBACK = 'No feedbacks added.';
const NO_LOWEST_BOUND_FEEDBACK = 'Lowest score bound feedback not specified.';
const SCORE_OUT_OF_RANGE = 'Score should be in range 0 through 100.';
private $feedback = array();
public static function instance($conf){
return new SWWWScoreFeedback($conf);
}
public function __construct($conf){
if(!$this -> insert($conf)){
throw new Exception(self::NO_FEEDBACK);
}
if( ! array_key_exists(0,$this -> feedback) ){
throw new Exception(self::NO_LOWEST_BOUND_FEEDBACK);
}
}
// add scores and feedbacks for them
private function insert($feedback){
foreach($feedback as $k => $v){
$ck = (int)str_replace('%','',$k);
if($ck >= 0 or $ck <= 100){
$this -> feedback[$ck] = $v;
}
}
return empty($this -> feedback) ? false : true;
}
// get feedback based on score provided
public function get($score){
$cscore = (int)str_replace('%','',$score);
$ck = null;
if($cscore >= 0 and $cscore <= 100){
if(array_key_exists($cscore,$this -> feedback)){
$ck = $cscore;
}else{
foreach($this -> feedback as $k => $f){
if($cscore > $k){
$ck = $k;
}else{
break;
}
}
}
}else{
throw new OutOfRangeException(self::SCORE_OUT_OF_RANGE);
}
return $this -> feedback[$ck];
}
}
SWWWScoreFeedback configuration
SWWWScoreFeedback should be configured by passing an associative array before it can be used. Keys of such an array are either numbers or percents (given as strings) in range from 0 to 100, with 0 the only key required. These keys are used to build intervals then. Values are strings representing feedback. There is no restriction on number of key / value pairs in the configuration array. Also, real numbers are as good as intergers for case.
$conf = array(
0 => 'Terrible!',
25 => 'You do not want to remember this!',
51 => 'You have passed, but only just.',
75 => 'Decent score!',
100 => 'Congratulations, this is a perfect score!'
);
In the above example, the intervals would be [0,25),[25,51),[51,75),[75,100),[100,100].
Once the configuration array is ready, you pass it to instance method of SWWWScoreFeedback and obtain an object.
$instance = SWWWScoreFeedback::instance($conf);
Getting feedback based on score
The object has just one public method called get. Get, when passed a number in range from 0 to 100 (or a percent) returns a string.
Here are some examples:
$instance -> get(0);
=> 'Terrible!'
$instance -> get('90.23%');
=> 'Decent score!'
$instance -> get(100);
=> 'Congratulations, this is a perfect score!'
Final thoughts
Regardless of programming language, mapping numbers to numbers, strings, functions or objects is a frequent task in computer programming. The need of mapping intervals crops up less frequently, still the technique is extremely useful for solving coding tasks like the one described in this text and many other, hence worth of spending some time on.
I hope the article was enjoyable and code in it simple enough to follow.
This post was updated on 09 Sep 2017 21:12:07
Tags:
php
Author, Copyright and citation
Author
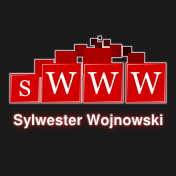
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2025 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. "Mapping numeric score to feedback message in PHP." From sWWW - Code For The Web . https://swww.com.pl//main/index/mapping-numeric-score-to-feedback-message-in-php
Add Comment