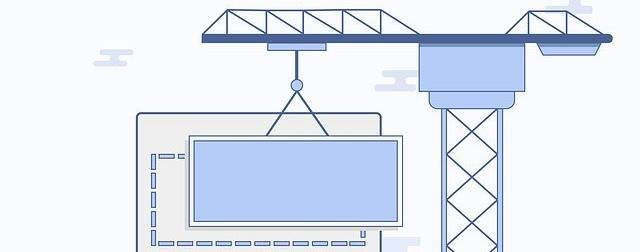
Developing for browser with mocha, chai and window node modules
Created:04 Aug 2020 23:21:09 , in Web development
Recently, when trying to write a bookmarklet for Firefox in JavaScript I faced a problem with testing DOM reliant operations in it. The bookmarklet was being written in Node environment and tested with Mocha and Chai. Since, node does not provide jsdom, I had to look for an extension which would add it. One such extensions, which provides global window object, available through NPM is Node module called Window.
This article shows how to test a jsdom reliant code using Mocha + Chai + Window.
Installing Mocha, Chai and Window
As mentioned above, Window module provides jsdom, for Mocha, it is a testing framework for Node. Chai is an assertion library which works well with Mocha.
Here is how to install all three on a Linux distribution like Debian using the command line: (for globally installed modules you will need root user privileges):,/p>
$ npm install mocha -g
$ npm install chai -g
$ npm install --save-dev window
Once installation process have competed, create a project folder and a src.js file in it. In the file paste the following code:
function DOMNode(document){
var node = null;
var document = document;
this.create = function(nodeName = 'DIV'){
node = document.createElement(nodeName);
return this;
}
this.setAttribute = function(attrName,attrValue){
node.setAttribute(attrName, attrValue);
return this;
}
this.innerHtml = function(html){
node.innerHTML = html;
return this;
}
this.addEventListener = function(eventName,callback){
node.addEventListener(eventName,callback);
return this;
}
this.appendChild = function(child){
node.appendChild(child);
return this;
}
this.get = function(){
return (function(){
return node;
})()
}
}
// export the function
module.exports.DOMNode = DOMNode;
Testing DOM operations with Mocha and Chai
To test code in src.js, create folder "test" and place file test.js in it.
In test.js past the following code:
// require the DOMNode function
const {DOMNode} = require('../src');
// require window
const Window = require('window');
const {document} = new Window;
// make chai assertions available
const assert = require('assert');
const expect = require('chai').expect;
describe('JSDom Tests', function() {
describe('#Dom Node', function() {
it('should enable node creation and manipulations', function() {
var node = new DOMNode(document);
// create new node
node.create();
// test setting attributes
node.setAttribute('id','stake');
// test adding callbacks
node.addEventListener('click',function(){return 'a';});
assert.equal(node.get().nodeName,'DIV');
// test getting attributes
assert.equal(node.get().getAttribute('id'),'stake');
// test inserting HTML
assert.equal(node.get().getAttribute('id'),'stake');
// test adding children
var child = new DOMNode(document);
child.create('SPAN');
assert.equal(child.get().nodeName,'SPAN');
// test appending a child
node.appendChild(child.get());
assert.equal(node.get().children[0].nodeName,'SPAN');
});
});
});
then on the command line:
cd test/ && mocha
to run your tests.
If everything went well, you should get a message, that all JSDom Tests have passed, which also tells you, that the setup is working and you are ready to focus on coding and testing on.
Conclusion
Window is not the only module which provides DOM API for node. Another is jsdom-global. Either of them provides functionality, which greatly helps with testing JavaScript code for browser being written in Node environment. Drop in jQuery and Lodash and you have a basis for a killer setup for building bookmarklets and other browser specific programs.
I hope you have found this article useful. If you have anything to add, leave a comment below.
This post was updated on 04 Aug 2020 23:34:54
Tags:
JavaScript , nodejs
Author, Copyright and citation
Author
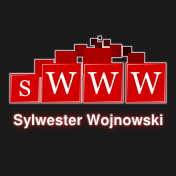
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2025 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. "Developing for browser with mocha, chai and window node modules." From sWWW - Code For The Web . https://swww.com.pl//main/index/developing-for-browser-with-mocha-chai-and-window-node-modules
Add Comment