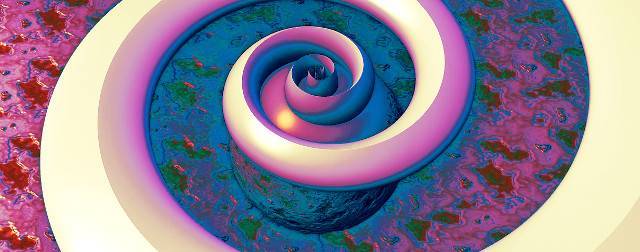
Square pyramidal numbers and implementing them in PHP
Created:19 Aug 2017 14:24:59 , in Maths
Square pyramidal numbers and implementing them in PHPImagine a couple of boxes standing on a table. In each of these boxes there is one or more balls. Boxes are sorted according to the number of balls they contain. When you look into these boxes, you notice, that in the first of them there is one ball, in the second - four, in the third - nine. Checking on you come to the conclusion that kth box contains k^2 balls.
The numbers constitute a sequence of positive perfect square numbers which is: 1,4,9, ..., k^2. with first term being 1.
Next you decide to take out balls from the first two boxes and arrange them in a pyramid with a square base. The sole ball from the first box goes on the top and 4 balls from the second box make up the base of the design.. Adding third level would require 9 balls from the third box.
Now, you want to know how many balls you would need to create a similar structure which has some greater number of levels. First level requires just one ball, for two-level design you need 5 balls (one for level 1 plus 4 for level 2). Coincidentally, 1 and 5 are the first two numbers of sequence of square pyramidal numbers. These numbers are sums of previous numbers in the sequence plus number of balls needed to add another level to the pyramid.
Hence, third square pyramidal number would be 14 (1 + 5 + 9 balls at the level 3 of the pyramid).
This information gives enough insight into how to build other square pyramidal numbers and also how to program building them.
Getting square pyramidal numbers recursively
Below is a recursive piece of PHP code which helps find a finite sequence of square pyramidal numbers or nth square pyramidal number:
/*
Class: SWWWSquarePyramidal
Description: Find finite sequence of square pyramidal numbers or nth square pyramidal number.
Author: Sylwester Wojnowski
WWW: wojnowski.net.pl
Methods:
squares - public static - find finite sequence of perfect square numbers
parameters:
n - int - number of square numbers to find
return - array
sequence - public static - find finite sequence of square pyramidal numbers
parameters:
n - int - number of square pyramidal numbers to find
return - array
number - public static - find kth pyramidal number
parameters:
k - int - kth square pyramidal number to return
return - int
*/
class SWWWSquarePyramidal{
// perfect squares sequence
public static function squares($n = 1){
if($n < 1){ return array(); }
$s = self::squares($n - 1);
$s[] = pow($n,2);
return $s;
}
// square pyramidal number series
public static function sequence($n,$s = null){
if($s === null) { $s = self::squares($n); }
if($n < 1){ return array(); }
if($n === 1){ return array(1); }
$series = self::sequence($n - 1,$s);
$series[] = $series[$n - 2] + $s[$n - 1];
return $series;
}
// kth square pyramidal number
public static function number($k){
return self::sequence($k)[$k - 1];
}
}
Use examples
Here are some use examples.
To obtain sequence of first five square pyramidal numbers run:
SWWWSquarePyramidal::sequence(5);
=> array(1,5,14,30,55),
Going back to the design, 1-level pyramid requires just one ball. 2,3,4 and 5-level pyramids - need 5,14,30,55 balls respectively.
To find out what the 10th square pyramidal number be:
SWWWSquarePyramidal::number(10);
=> 385
It looks like, 385 balls are needed to build a 10-level pyramid with square base.
This number can also be found using a closed-form expression. The formula for nth square pyramidal number is n*(n+1)(2n+1)/6.
Substitute 10 for n to get the above result.
The PHP class can also be used to output sequence of perfect square numbers:
SWWWSquarePyramidal::squares(5);
=> array(1,4,9,16,25)
Final thoughts
Taking a close look at square pyramidal numbers helps in understanding how sequences and series work and how they can be applied to other real-life situations. As for coding part, building a program capable of outputting these numbers is a nice exercise in recursive programming.
I hope you've found the article instructive and code given easy to understand.
This post was updated on 09 Sep 2017 10:17:23
Author, Copyright and citation
Author
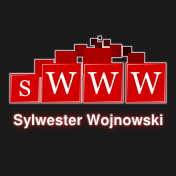
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2024 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. "Square pyramidal numbers and implementing them in PHP." From sWWW - Code For The Web . https://swww.com.pl//main/index/square-pyramidal-numbers-and-implementing-them-in-php
Add Comment