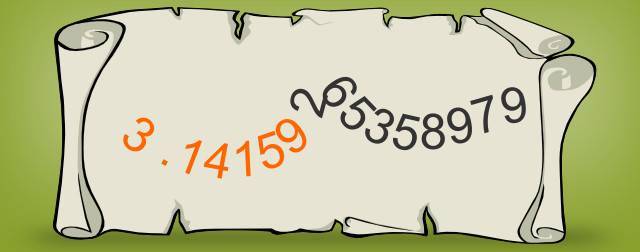
Formatting array of numbers recursively in PHP
Created:12 Aug 2017 15:57:38 , in Maths
If you have ever tried to obtain a formatted value in PHP, it is likely you did so with one of printf, sprintf of vsprintf functions. In general, these utilities accept a formatting string and one or more arguments. php.net has some good examples and descriptions on how they work, how to build formatting strings, and what to expect as the return value from each of them.
The goal of this article is to extend usefulness of the functions by providing (a recursive) method for formatting arrays. At the time of writing the function I was primarily interested in numbers. So, this text is about formatting arrays of numbers. However, the method provided is generic enough to let you format arrays of whatever objects you wish.
ArrayExtensions::format method
My formatting utility method is called "format". It is a public static function in ArrayExtensions class.
Here is the code ArrayExtensions class consists of:
/*
Class: ArrayExtensions
Description: Provides recursive format function
Author: Sylwester Wojnowski
WWW: wojnowski.net.pl
Methods:
format - format array of objects according to a formatting string
$format - string - formatting string as described for sprintf PHP function
$subject - array of objects
return array - array of formatted objects
*/
class ArrayExtensions{
public static function format($format,$subject,$n = null){
$n = ( $n !== null ) ? $n : count($subject);
// subject is empty = nothing to format
if($n === 0){return $subject;}
// format first item
if($n === 1){
return array( sprintf($format,$subject[ $n - 1 ]) );
}
// get to the first item
$formatted = self::format($format,$subject,$n - 1);
// format next item
$formatted[] = sprintf($format,$subject[ $n - 1 ]);
return $formatted;
}
}
Some use examples
Here are use examples. In each case case an array of strings is returned.
Turn array of integers into array of floating-point numbers:
ArrayExtensions::format('%.2f',array(1,2,3))
=> array('1.00','2.00','3.00'),
Display first six terms of sequence obtained using formula a(n) = 1 / ((-1)^(n-1)*n^2) formatted as floating-point numbers, each with 3 decimal digits:
ArrayExtensions::format('%.3f',array(1,-1/4,1/9,-1/16,1/25,-1/36,1/49))
=>array('1.000','-0.250','0.111','-0.062','0.040','-0.028','0.020')
I guess these two quick examples give enough insight in how the ArrayExtensions::format works, and how to format arrays of numbers with it.
Final thoughts
ArrayExtensions::format is a recursive method, but something of very similar functionality can be written using a simple loop. I used recursion due to the latter being more universal tool (just a subjective view of mine). This universalism is hardly visible for simple cases like this one but proves crucial when one moves from dealing with arrays to traversing trees.
As usual, I hope you'll find the code interesting and, above all, useful.
This post was updated on 12 Aug 2017 18:27:51
Author, Copyright and citation
Author
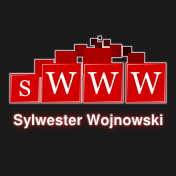
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2025 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. "Formatting array of numbers recursively in PHP." From sWWW - Code For The Web . https://swww.com.pl//main/index/formatting-array-of-numbers-recursively-in-php
Add Comment