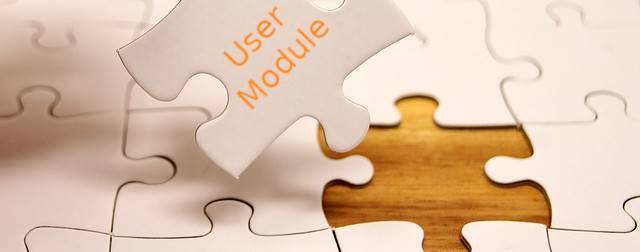
Building a module for CodeIgniter 4
Created:28 Jan 2022 12:34:11 , in Web development
Once you are somewhat familiar with basics of CodeIgniter 4 and the MVC pattern it uses, it is time to move on to building modules for the framework. Modules are chunks of functionality you write once and reuse in your current and subsequent projects. Depending on your needs you might need a blog module, a gallery module or a social media module. The idea is, each of these modules should require minimal changes to the code as they are reused in your new project. Moreover all required changes should be made in module's configuration files.
In this article I look how to set up a basic User module for Codeigniter 4. The setup can be split up into two parts. First is about building a basic module structure, second about connecting the module to your wider application.
Building a User module structure
A module in CodeIgniter 4 consists of a few parts. They are a controller, a model, view(s), libraries and config files.
So, lets craete these. In your project root directory (the one with app and system directories), create a folder called modules. In the folder create folder called User. Enter the folder and create folders Controllers, Config, Libraries, Models and Views.
Switch to Controllers directory and create file Users.php. It should contain contents as follows:
namespace Modules\User\Controllers;
use App\Controllers\BaseController;
use Modules\User\Models\User;
class Users extends BaseController{
protected $configValue1 = '';
public function initController($request, $response, $logger){
parent::initController($request, $response, $logger);
$config = config('\Modules\User\Config\User');
$this -> configValue1 = $config -> configValue1;
}
public function login(){
$data = [];
return view('Modules\User\Views\login',$data);
}
}
Now, switch to Models directory and create file called User.php with contents like this:
namespace Modules\User\Models;
use CodeIgniter\Model;
class User extends Model {
}
Next, switch to Views folder and crate file called login.php. This is your first view for this module. You can add some HTML in it.
Finally, let's create configuration files for this module, one with general configuration values and one with routes. Switch to Config directory and create file User.php with content as follows:
namespace Modules\User\Config;
use CodeIgniter\Config\BaseConfig;
class User extends BaseConfig{
// add your module configuration here
public $configValue1 = 'some configuration value here';
}
Now, in Config directory create file called Routes with contents like:
$routes->get('/user/login$', '\Modules\User\Controllers\Users::login');
$routes->post('/user/login$', '\Modules\User\Controllers\Users::login');
A basic file/directory structure for User module is complete. Now, it is time to make the module available to the rest of your CodeIgniter 4 application.
Connecting User module to application
To make your module available to your application you need two steps. Firstly, add modules directory to psr4 array in app/Config/Autoload.php. The array should look like this:
public $psr4 = [
APP_NAMESPACE => APPPATH, // For custom app namespace
'Config' => APPPATH . 'Config',
// add Modules namespace
'Modules' => ROOTPATH. 'modules',
];
In step two open app/Config/Routes.php and add entry like this:
// include User module
if (file_exists(ROOTPATH . 'modules/User/Config/Routes.php')){
require ROOTPATH . 'modules/User/Config/Routes.php';
}
This ensures your application has access to routing directives specified in your modules Routes.php file.
At this point you should be able to access route like /user/login without an error and see stuff your placed in your modules/User/Views/login.php file.
Conclusion
Building a CodeIgniter 4 module can be a bit complicated, especially taking into account amount of namespacing involved, but this short guide should give you enough information to complete these sort of tasks quickly and successfully.
Tags:
CodeIgniter , php
Author, Copyright and citation
Author
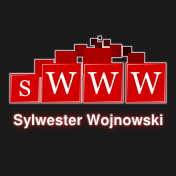
Author of the this article - Sylwester Wojnowski - is a sWWW web developer. He has been writing computer code for the websites and web applications since 1998.
Copyrights
©Copyright, 2024 Sylwester Wojnowski. This article may not be reproduced or published as a whole or in parts without permission from the author. If you share it, please give author credit and do not remove embedded links.
Computer code, if present in the article, is excluded from the above and licensed under GPLv3.
Citation
Cite this article as:
Wojnowski, Sylwester. "Building a module for CodeIgniter 4." From sWWW - Code For The Web . https://swww.com.pl//main/index/building-a-module-for-codeigniter-4
Add Comment